At Make Do we have been lucky enough to work with several WordPress VIP agencies, and have built plugins that work in their development environments.
This has given us an advantage over other Technical WordPress agencies aimed at SME businesses, as we have adopted the working practices of our VIP partners, offering an enterprise level development service.
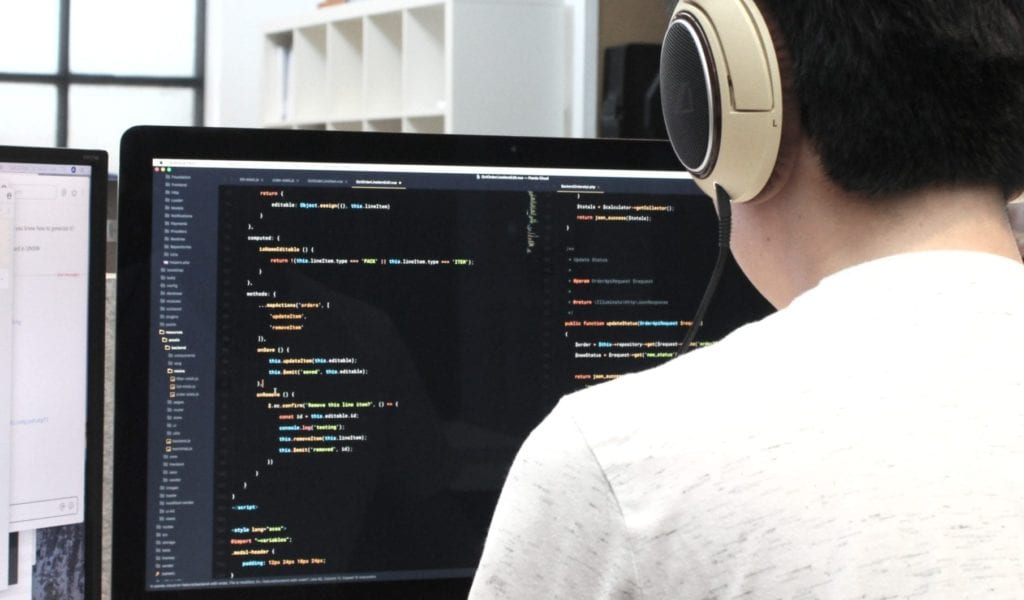
Here is a quick guide to our approach to building plugins at VIP agency level.
1. Get to Grips with the Command Line (CLI)
Most professional agencies do not use tools that provide a UI, as it is much quicker and effective to know the native commands for many tools, with others not even providing a UI.
Tools you may need to use with the command line include (but are certainly not limited to):
- wp cli
- git
- npm
- yarn
- brew
2. Version Control (such as Git) is a Must
Branching and Merging are an absolute must when building a plugin for a VIP agency. You must have a good understanding of:
- Creating a new branch
- Selectively Adding and Commit-ing code to a branch
- Creating a Pull Request
- Merging a branch to another branch
- Reviewing a Pull Request
- Merging a Pull Request
- Rebasing a branch
Along the way you will likely need to use more complex concepts such as cherry picking, and undoing commits.
3. Gain a Deep Understanding of WordPress Hooks, Filters and Helper Functions
Do things the ‘WordPress way’. Avoid third party libraries, or recreating functions that WordPress does anyway.
Many times I have seen inexperienced agencies pull in the full Laveral framework, or have a file full of escaping or SQL access functions, when they could have just used the native WordPress functions.
If you want to write a plugin at agency level, you need to know WordPress, and anything you don’t know, be prepared to search the documentation or the codebase first, before you write something completely new.
4. Structure: Use PHP Classes and Namespaces
You may not know, but there are multiple ways to write code for WordPress, and the majority of the examples online use the functional approach, eg:
function namespace_my_function() {
// Do Code.
}
add_action( 'init', 'namespace_my_function' );
This works absolutely fine, but for the most part, using PHP classes and namespaces saves you having to namespace your functions and brings far more structure to your plugin.
Lets look at that example from a Class based approach:
namespace company_name\plugin_name;
class Main {
public function run() {
add_action( 'init', array( this, 'my_function' ) );
}
public function my_function() {
// Do Code.
}
}
$main = new Main();
$main->run();
This is a very basic example, and using Classes like this allows you to take advantage of many Object Oriented Programming (OOP) patterns, including using Singleton and Factory patterns in your code.
5. Learn JavaScript Deeply
The new block editor for WordPress (Gutenberg) introduced a JavaScript centric way of programming for the WordPress editor. To get up and running with this you need to be familiar with concepts such as JavaScript ESNext and React.
In the near future, the majority of the WordPress editor will be JavaScript based like this, so if you have not yet delved into the world of JavaScript programming, now is the time.
6. Escape All the Things!
Security should be at the forefront of everything you write. As well as the usual checks for Nonce fields in your code you should be using the escaping functions in WordPress to ensure that every input and every output is safe before it goes into, or comes out of the Database.
For example, you may need to escape output with:
esc_html()
– To escape HTML in the outputesc_attr()
– To escape attributes that are outputtedesc_url()
– To escape URLswp_kses()
– To escape HTML (pass in the allowed tags as an array)
And input may be escaped with the following:
sanitize_text_field()
– To escape GET or POST requests from input fields.sanitize_textarea_field()
– To escape GET or POST requests fromt extareas.
For more information, visit the section in the WordPress handbook all about Escaping.
7. Be Able to Take Constructive Criticism
When I first started providing work for our VIP partners, my work was sent back to me many times do re-do, or make amendments to.
Initially I got a little protective over my work, but I soon realised what the feedback was doing, which was making me a far better developer than I ever was before I started taking on these projects.
Eventually I submitted my work confident it wouldn’t come back with any feedback, and if it did come back I welcomed it, as it meant I would learn something new from my peers, who at the time had been doing this much longer, and to a much higher standard than I had.
8. Be Patient, and Be Prepared to Explain
When working with VIP agencies, you will find that a lot of time you are working with international branches of the agency, or international clients. This means you inevitably come across language barriers.
This can be frustrating, as finding the words to explain a complex non-tangible concept (as code often is) across a language barrier can be tricky, however patience and explanation can often ease this difficulty.
Need Some Custom Plugin Help?
Finally: Learn, Expand and Challenges Yourself
As a heavily Technical WordPress Team our agency is always looking for ways to get better at what we do and at different ways we can deliver bespoke technical WordPress solutions.
The experience gained building custom WordPress plugins for all of our clients goes into every future project we build and we always aim to challenge our engineers to do more!